Csv Error Invalid Csv File Format. Error Reading Row
The CSV (Comma Separated Values) format is quite popular for storing data. A big number of datasets are present equally CSV files which can be used either straight in a spreadsheet software like Excel or can exist loaded up in programming languages like R or Python. Pandas dataframes are quite powerful for treatment two-dimensional tabular data. In this tutorial, nosotros'll expect at how to read a csv file as a pandas dataframe in python.
How to read csv files in python using pandas?
The pandas read_csv()
function is used to read a CSV file into a dataframe. It comes with a number of different parameters to customize how you'd similar to read the file. The following is the general syntax for loading a csv file to a dataframe:
import pandas as pd df = pd.read_csv(path_to_file)
Here, path_to_file
is the path to the CSV file you lot desire to load. It tin exist whatsoever valid string path or a URL (encounter the examples below). It returns a pandas dataframe. Permit'south look at some of the unlike apply-cases of the read_csv()
office through examples –
Examples
Before we go along, allow'south get a sample CSV file that we'd be using throughout this tutorial. We'll be using the Iris dataset which you tin download from Kaggle. Here's a snapshot of how it looks when opened in excel:
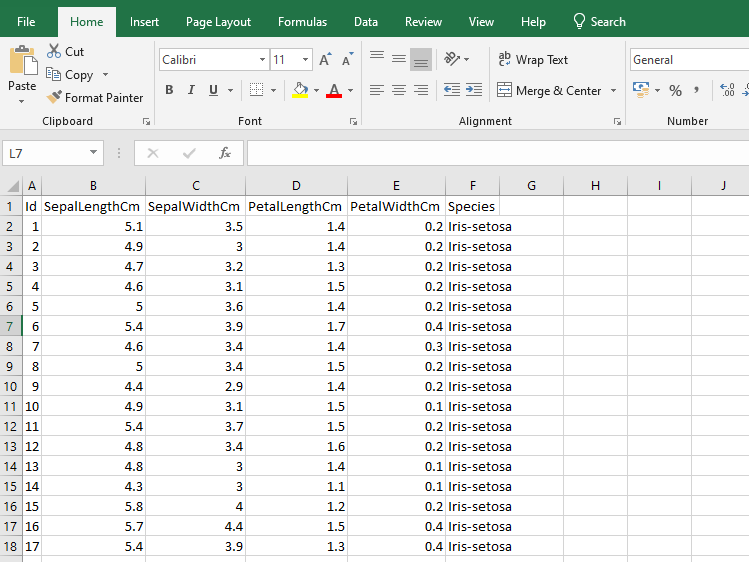
1. Read CSV from its location on your machine
To read a CSV file locally stored on your auto pass the path to the file to the read_csv()
function. You tin can pass a relative path, that is, the path with respect to your current working directory or you lot can pass an absolute path.
# read csv using relative path import pandas as pd df = pd.read_csv('Iris.csv') print(df.caput())
Output:
Id SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species 0 one 5.1 3.v i.4 0.2 Iris-setosa 1 2 4.9 3.0 1.4 0.2 Iris-setosa 2 3 4.7 3.2 i.3 0.ii Iris-setosa 3 4 4.6 3.1 i.5 0.ii Iris-setosa 4 5 five.0 3.half-dozen i.iv 0.2 Iris-setosa
In the to a higher place case, the CSV file Iris.csv
is loaded from its location using a relative path. Here, the file is nowadays in the current working directory. Y'all can also read a CSV file from its absolute path. See the example below:
# read csv using absolute path import pandas as pd df = pd.read_csv(r"C:\Users\piyush\Downloads\Iris.csv") impress(df.head())
Output:
Id SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species 0 1 5.1 3.5 1.4 0.2 Iris-setosa ane 2 four.9 three.0 1.four 0.2 Iris-setosa 2 3 four.vii 3.2 1.3 0.two Iris-setosa iii 4 iv.6 three.1 ane.5 0.2 Iris-setosa iv five 5.0 3.vi 1.4 0.2 Iris-setosa
Here, the same CSV file is read from its absolute path.
2. Read CSV from a URL
Yous can besides read a CSV file from its URL. Pass the URL to the read_csv()
office and it'll read the corresponding file to a dataframe. The Iris dataset can likewise be downloaded from the UCI Machine Learning Repository. Permit'southward use their dataset download URL to read information technology as a dataframe.
import pandas equally pd df = pd.read_csv("https://archive.ics.uci.edu/ml/car-learning-databases/iris/iris.information") df.head()
Output:
5.1 3.5 1.4 0.2 Iris-setosa 0 4.9 three.0 1.4 0.2 Iris-setosa 1 iv.7 iii.two 1.3 0.ii Iris-setosa 2 4.half dozen three.1 1.5 0.2 Iris-setosa 3 5.0 3.vi 1.four 0.2 Iris-setosa 4 five.four iii.9 one.seven 0.4 Iris-setosa
You lot can see that the read_csv()
function is able to read a dataset from its URL. It is interesting to note that in this particular data source, we practise not have headers. The read_csv()
role infers the header by default and here uses the outset row of the dataset equally the header.
iii. Read a CSV file without a header
In the above example, you saw that if the dataset does not have a header, the read_csv()
role infers it by itself and uses the first row of the dataset every bit the header. Yous can alter this behavior through the header
parameter, pass None
if your dataset does not have a header. You tin also pass a custom list of integers as a header.
import pandas as pd df = pd.read_csv("https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.data", header=None) df.head()
Output:
0 1 2 3 four 0 5.1 iii.5 one.4 0.2 Iris-setosa one four.nine three.0 i.4 0.2 Iris-setosa two 4.seven 3.2 1.3 0.2 Iris-setosa three 4.6 iii.1 i.5 0.two Iris-setosa 4 5.0 3.6 1.4 0.ii Iris-setosa
In the in a higher place example, we laissez passer header=None
to the read_csv()
role since the dataset did not take a header.
iv. Read a CSV file and give custom column names
You tin requite custom column names to your dataframe when reading a CSV file using the read_csv()
function. Laissez passer your custom column names equally a list to the names
parameter.
import pandas as pd df = pd.read_csv("https://archive.ics.uci.edu/ml/machine-learning-databases/iris/iris.information", names = ['SepalLengthCm', 'SepalWidthCm', 'PetalLengthCm', 'PetalWidthCm', 'Species']) impress(df.head())
Output:
SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species 0 5.1 3.5 1.iv 0.2 Iris-setosa 1 four.9 iii.0 one.four 0.2 Iris-setosa ii four.seven 3.2 1.iii 0.2 Iris-setosa iii 4.6 iii.1 1.five 0.two Iris-setosa 4 5.0 3.vi 1.four 0.2 Iris-setosa
v. Read CSV with a column as index
You lot tin too use a column as the row labels of the dataframe. Laissez passer the cavalcade name to the index_col
parameter. Going back to the Iris.csv
we downloaded from Kaggle. Here, we utilize the Id
columns every bit the dataframe index.
# read csv with a column equally index import pandas every bit pd df = pd.read_csv('Iris.csv', index_col='Id') impress(df.head())
Output:
SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species Id 1 5.1 3.5 ane.4 0.2 Iris-setosa 2 four.ix 3.0 1.4 0.2 Iris-setosa 3 iv.7 3.2 1.3 0.2 Iris-setosa four four.6 3.ane 1.5 0.2 Iris-setosa 5 5.0 3.6 1.4 0.2 Iris-setosa
In the higher up example, you lot can run into that the Id
column is used as the row index of the dataframe df
. You can also pass multiple columns equally list to the index_col
parameter to be used equally row alphabetize.
six. Read simply a subset of columns of a CSV
Yous tin can also specify the subset of columns to read from the dataset. Pass the subset of columns you want as a list to the usecols
parameter. For example, let'southward read all the columns from Iris.csv
except Id
.
# read csv with a column as alphabetize import pandas every bit pd df = pd.read_csv('Iris.csv', usecols=['SepalLengthCm', 'SepalWidthCm', 'PetalLengthCm', 'PetalWidthCm', 'Species']) print(df.head())
Output:
SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species 0 five.1 three.5 1.4 0.two Iris-setosa 1 4.9 iii.0 1.4 0.2 Iris-setosa 2 4.7 3.two 1.3 0.2 Iris-setosa 3 four.6 3.1 1.5 0.2 Iris-setosa 4 v.0 3.half dozen 1.4 0.2 Iris-setosa
In the above example, the returned dataframe does non have an Id
cavalcade.
vii. Read only the first n rows of a CSV
You can too specify the number of rows of a file to read using the nrows
parameter to the read_csv()
part. Specially useful when you want to read a small segment of a large file.
# read csv with a column as index import pandas every bit pd df = pd.read_csv('Iris.csv', nrows=3) impress(df.head())
Output:
Id SepalLengthCm SepalWidthCm PetalLengthCm PetalWidthCm Species 0 i 5.1 three.v 1.4 0.2 Iris-setosa 1 2 four.ix 3.0 1.4 0.2 Iris-setosa ii iii 4.7 3.2 1.3 0.2 Iris-setosa
In the above case, we read only the first three rows of the file Iris.csv
.
These are merely some of the things you can do when reading a CSV file to dataframe. Pandas dataframes also provide a number of useful features to manipulate the data once the dataframe has been created.
With this, nosotros come up to the finish of this tutorial. The code examples and results presented in this tutorial accept been implemented in a Jupyter Notebook with a python (version three.8.3) kernel having pandas version i.0.v
Subscribe to our newsletter for more than informative guides and tutorials.
Nosotros do not spam and you tin opt out any fourth dimension.
Source: https://datascienceparichay.com/article/read-csv-files-using-pandas-with-examples/
0 Response to "Csv Error Invalid Csv File Format. Error Reading Row"
Postar um comentário